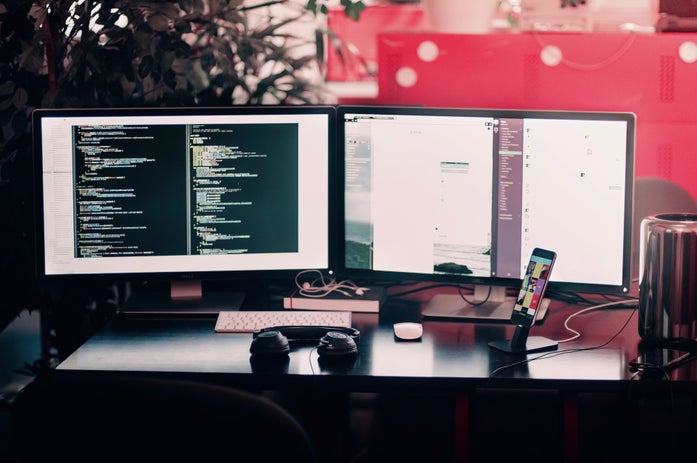
If you would like to build your own blockchain, you need code to do it. In this blog, I will provide you with a some general code that you can use to build your own blockchain network. Another important component of a blockchain is a consensus mechanism. In this blog, I will also provide general code of the Proof of Work consensus algorithm.
Here's an example of a simple blockchain implementation in Python that uses a consensus algorithm based proof-of-work:
import hashlib
import time
class Block:
def __init__(self, data, previous_hash):
self.timestamp = time.time()
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def calculate_hash(self):
hash_data = str(self.timestamp) + str(self.data) + str(self.previous_hash)
return hashlib.sha256(hash_data.encode('utf-8')).hexdigest()
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
self.difficulty = 4
def create_genesis_block(self):
return Block("Genesis Block", "0")
def get_latest_block(self):
return self.chain[-1]
def add_block(self, new_block):
new_block.previous_hash = self.get_latest_block().hash
new_block.hash = new_block.calculate_hash()
self.chain.append(new_block)
def is_chain_valid(self):
for i in range(1, len(self.chain)):
current_block = self.chain[i]
previous_block = self.chain[i-1]
if current_block.hash != current_block.calculate_hash():
return False
if current_block.previous_hash != previous_block.hash:
return False
return True
def mine(self, new_block):
while True:
if new_block.hash[:self.difficulty] == '0'*self.difficulty:
self.add_block(new_block)
break
else:
new_block.timestamp = time.time()
new_block.hash = new_block.calculate_hash()
blockchain = Blockchain()
# create and add some blocks to the chain
block1 = Block("Data for block 1", blockchain.get_latest_block().hash)
blockchain.mine(block1)
block2 = Block("Data for block 2", blockchain.get_latest_block().hash)
blockchain.mine(block2)
block3 = Block("Data for block 3", blockchain.get_latest_block().hash)
blockchain.mine(block3)
# print out the blockchain to verify it's valid
for block in blockchain.chain:
print("Hash:", block.hash)
print("Data:", block.data)
print("Previous Hash:", block.previous_hash)
print("Timestamp:", block.timestamp)
print("----------------------")
In this example, we define a Block class to represent individual blocks in the blockchain, and a Blockchain class to manage the chain of blocks.
The Blockchain class includes a mine() method that implements the proof-of-work consensus algorithm. In this algorithm, a new block is added to the chain by repeatedly hashing it with different nonce values until a hash is found that meets a certain difficulty requirement (in this case, a certain number of leading zeroes).
To add a block to the chain, we create a new Block instance with the relevant data and the hash of the previous block as the previous_hash argument. We then call the mine() method with the new block as an argument to add it to the chain.
Finally, we print out the contents of the blockchain to verify that it's valid.
Reactie plaatsen
Reacties