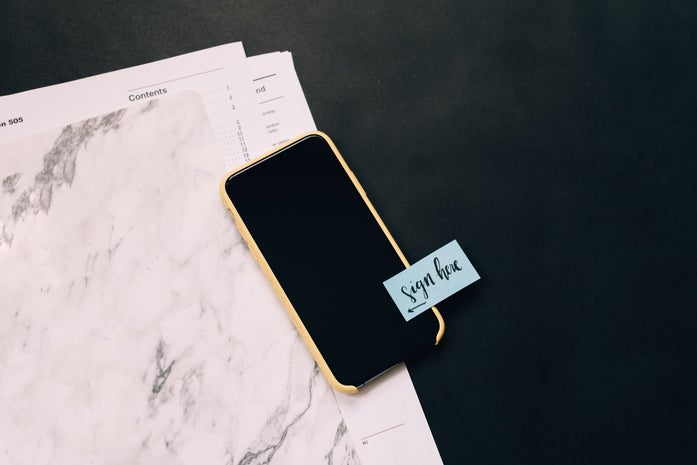
The digital signature is the main component of blockchain technology. Without a digital signature, blockchain technology wouldn't be safe to use. In this blog, I will provide you with a sample code to implement in your own blockchain. Digital signature isn't only used for blockchain technology but can used in various applications where transactions is a use case.
Digital signatures are an important component of blockchain technology because they provide a way to verify the authenticity and integrity of transactions and other data on the blockchain. Specifically, digital signatures allow participants on the blockchain to prove that they are the legitimate owner of a particular asset or identity, and that they have authorized specific actions or transactions related to that asset or identity.
In a blockchain network, every transaction is signed by the sender using their private key, which is then verified by the network using the corresponding public key. This ensures that only the owner of the private key can initiate transactions, and that all transactions are tamper-proof and cannot be altered by anyone else on the network.
Digital signatures are also important in blockchain because they help to prevent fraud and other malicious activities on the network. For example, if a hacker were to attempt to modify a transaction or alter the ledger in some way, the digital signature would no longer be valid and the network would reject the transaction.
Overall, digital signatures are a critical component of blockchain technology, providing a way to verify ownership, ensure the integrity of transactions and data, and prevent fraud and other malicious activities.
Here is some example code in Python for generating and verifying a digital signature using the ECDSA (Elliptic Curve Digital Signature Algorithm) algorithm:
import hashlib
import ecdsa
# Generate a new private key
sk = ecdsa.SigningKey.generate(curve=ecdsa.SECP256k1)
# Generate the corresponding public key
vk = sk.get_verifying_key()
# Create a message to sign
message = b"Hello, world!"
# Hash the message using SHA-256
message_hash = hashlib.sha256(message).digest()
# Sign the message hash using the private key
signature = sk.sign(message_hash)
# Verify the signature using the public key
try:
vk.verify(signature, message_hash)
print("Signature is valid!")
except ecdsa.BadSignatureError:
print("Signature is invalid.")
In this example, we first generate a new private key using the SigningKey.generate() method from the ecdsa library. We then derive the corresponding public key using the get_verifying_key() method.
Next, we create a message to sign (in this case, the bytes "Hello, world!"). We hash the message using the SHA-256 algorithm to produce a message hash.
We then use the private key to sign the message hash using the sign() method. This produces a signature, which is a string of bytes that can be sent along with the message.
Finally, we verify the signature using the public key and the verify() method. If the signature is valid, the verify() method will return True, indicating that the message was indeed signed by the owner of the private key. Otherwise, it will raise a BadSignatureError indicating that the signature is invalid.
Reactie plaatsen
Reacties